Sticky CTA
Display a call to action that follows the viewport
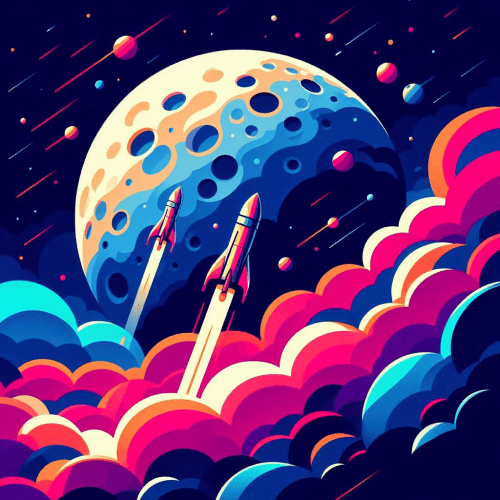
Get access to hundreds of courses
Use the StickyCta
component to display a fixed call to action that follows the viewport on scroll. They can increase conversion rate by improving visibility. Use them for promotional key actions, like “Buy Now”, “Subscribe”, or to direct users to a landing page. To get started, pass a cta
prop to the component:
---const cta = { text: 'Get Started ->', theme: 'secondary', href: '#'}---
<StickyCta cta={cta}> Unlock exclusive content</StickyCta>
You can use the StickyCta
component with a single cta
prop that defines the props for a CTA button. You can use any valid ButtonProps
type to customize your CTA, along with a text
and icon
prop to set the button’s text and icon. Inside your component, you can define your call to action, along with any other element:
Get access to hundreds of courses
---import { Icon } from 'webcoreui/astro'---
<StickyCta cta={cta}> <Icon type="github" /> <div> <strong>Unlock exclusive content</strong><br /> <span>Get access to hundreds of courses</span> </div></StickyCta>
Thumbnails
To improve the appearance of your sticky CTA, you can optionally add thumbnails. Use the img
prop to describe your thumbnail:
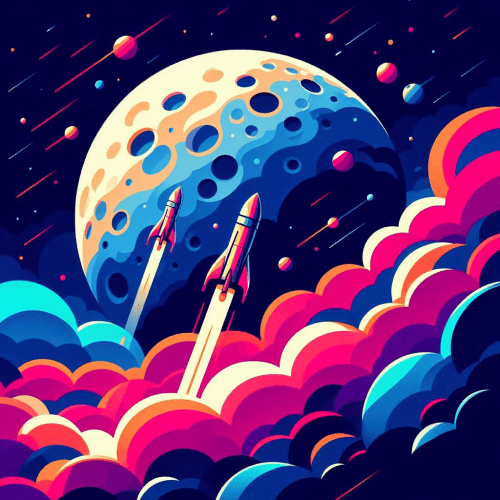
Get access to hundreds of courses
---const cta = { ... }const img = { src: '/img/thumbnail.png', alt: 'Thumbnail', width: 60, height: 60}---
<StickyCta cta={cta} img={img}> <div> <strong>Unlock exclusive content</strong><br /> <span>Get access to hundreds of courses</span> </div></StickyCta>
When using thumbnail images, you can optionally add a lazy
prop and set it to true
to lazy-load the image.
Themes
The StickyCTA
component comes with 6 different themes out of the box. To set a theme, use the theme
prop:
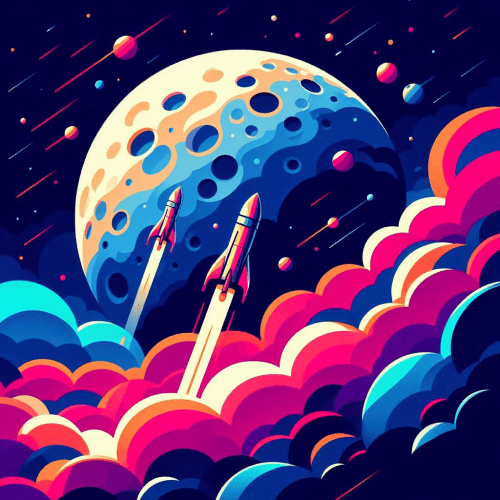
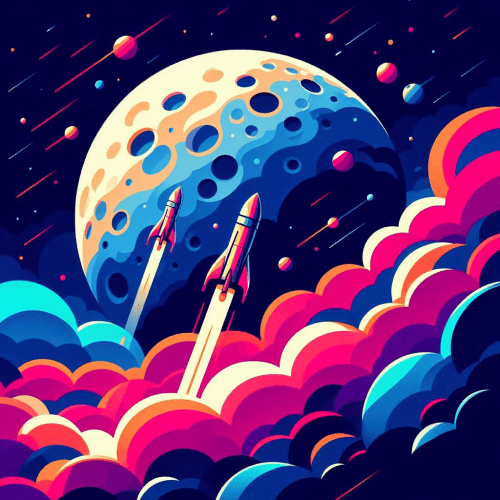
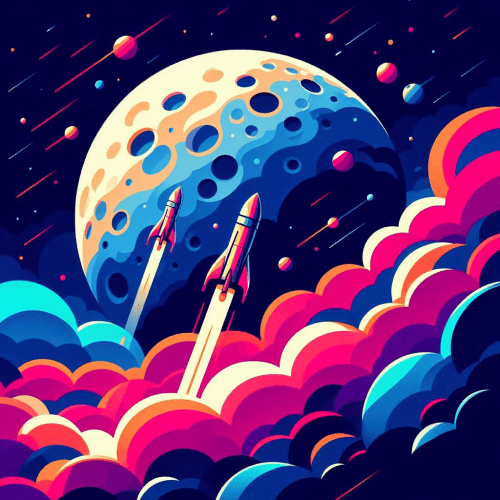
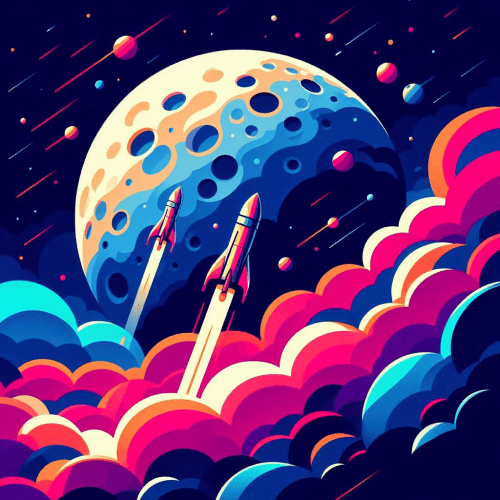
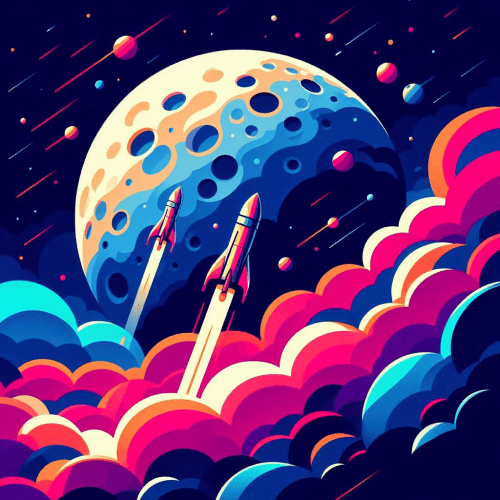
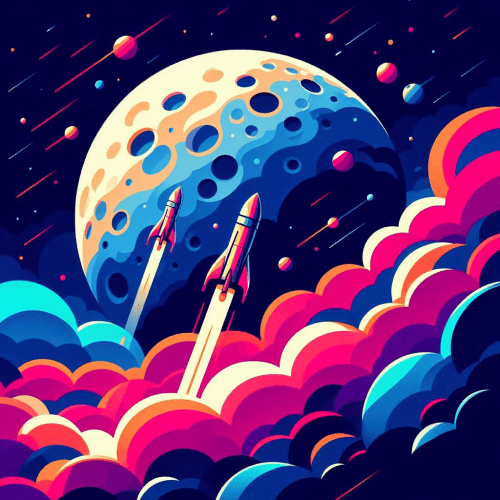
<StickyCta cta={cta} img={img} theme="secondary">...</StickyCta><StickyCta cta={cta} img={img} theme="info">...</StickyCta><StickyCta cta={cta} img={img} theme="success">...</StickyCta><StickyCta cta={cta} img={img} theme="warning">...</StickyCta><StickyCta cta={cta} img={img} theme="alert">...</StickyCta>
Note that you can separately style the theme of the CTA button using the cta.theme
prop. For available button themes, see the API documentation of the Button
component.
Trigger Actions
By default, the sticky CTA will float at the bottom of the viewport. This behavior can be customized, and you can use the following props to trigger the sticky CTA based on when an element enters or leaves the viewport:
showBefore
: Shows the sticky CTA before an element appears in the viewport.showAfterEnter
: Shows the sticky CTA after an element enters the viewport.showAfter
: Shows the sticky CTA after an element leaves the viewport.
<Alert theme="info" className="sticky-trigger"> Scroll past this element to trigger a sticky CTA.</Alert>
<StickyCta cta={...} img={...} showAfter=".sticky-trigger">...</StickyCta>
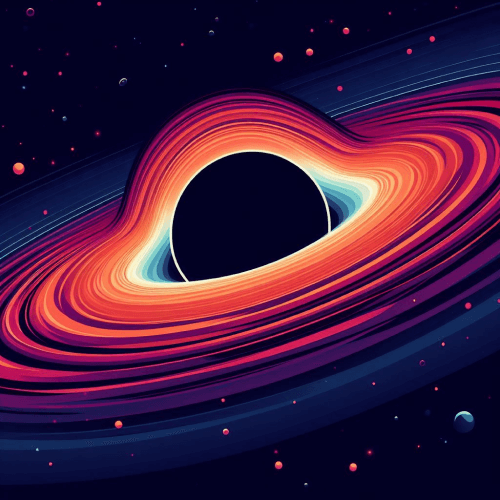
API
type StickyCtaProps = { img?: { src: string alt: string width: number height: number lazy?: boolean } cta: ({ text: string icon?: string } & ButtonProps) theme?: 'secondary' | 'info' | 'success' | 'warning' | 'alert' showBefore?: string showAfterEnter?: string showAfter?: string rootMargin?: string className?: string id?: string}
Button
component for the ButtonProps
type. Prop | Purpose |
---|---|
img | Sets a small thumbnail image for the CTA element. |
img.src | Sets the path for the image. |
img.alt | Sets an alternate text for the image. |
img.width | Sets the width of the image. |
img.height | Sets the height of the image. |
img.lazy | Set to true to enable lazy loading for the image. |
cta | Sets the CTA button. |
cta.text | Sets the label for the CTA. |
cta.icon | Sets an icon for the CTA. You can pass SVG strings, or an icon type for Astro components. |
theme | Sets the theme of the sticky CTA. |
showBefore | Shows the sticky CTA before it reaches the target selector. |
showAfterEnter | Shows the sticky CTA after the target selector enters the viewport. |
showAfter | Shows the sticky CTA after the target selector exits the viewport. |
rootMargin | Sets a custom root margin for the trigger element. |
className | Pass additional CSS classes for the StickyCta component. |
id | Specify a unique ID for the StickyCta component. |