Layout
Structure your page with a single component
Use the Layout
component to structure and organize content on your page. They can help you speed up your development process by providing a single component that you can use to quickly set up new pages. They’re responsive, and can help you achieve a consistent design across different pages.
---import Layout from '@blocks/Layout.astro'
const seo = { ... }const menu = { ... }const footer = { ... }---
<Layout seo={seo} menu={menu} footer={footer}> Your main content</Layout>
To customize your layout, you can set the following three props:
seo
: Use to configure metadata in thehead
tag. It expectsSEOProps
as a list of valid properties.menu
: Use to configure your main navigation. It expects theMenuProps
format for the properties.footer
: Use to configure your footer. It expects theFooterProps
format for the properties.
---const seo = { title: 'Your title', url: 'https://webcoreui.dev/', description: 'A short description for your page', faviconUrl: 'https://webcoreui.dev/favicon.ico'}
const menu = { logo: { html: '<svg ... />' }}
const footer = { logo: { html: '<svg ... />' }, columns: [ { title: 'Sitemap', items: [ { href: '/', name: 'Home' } ] } ], subText: `© ${new Date().getFullYear()} Webcore.`}---
Layout
component in our Templates library. Slots
You can use the following named slots to insert content into different places of your layout:
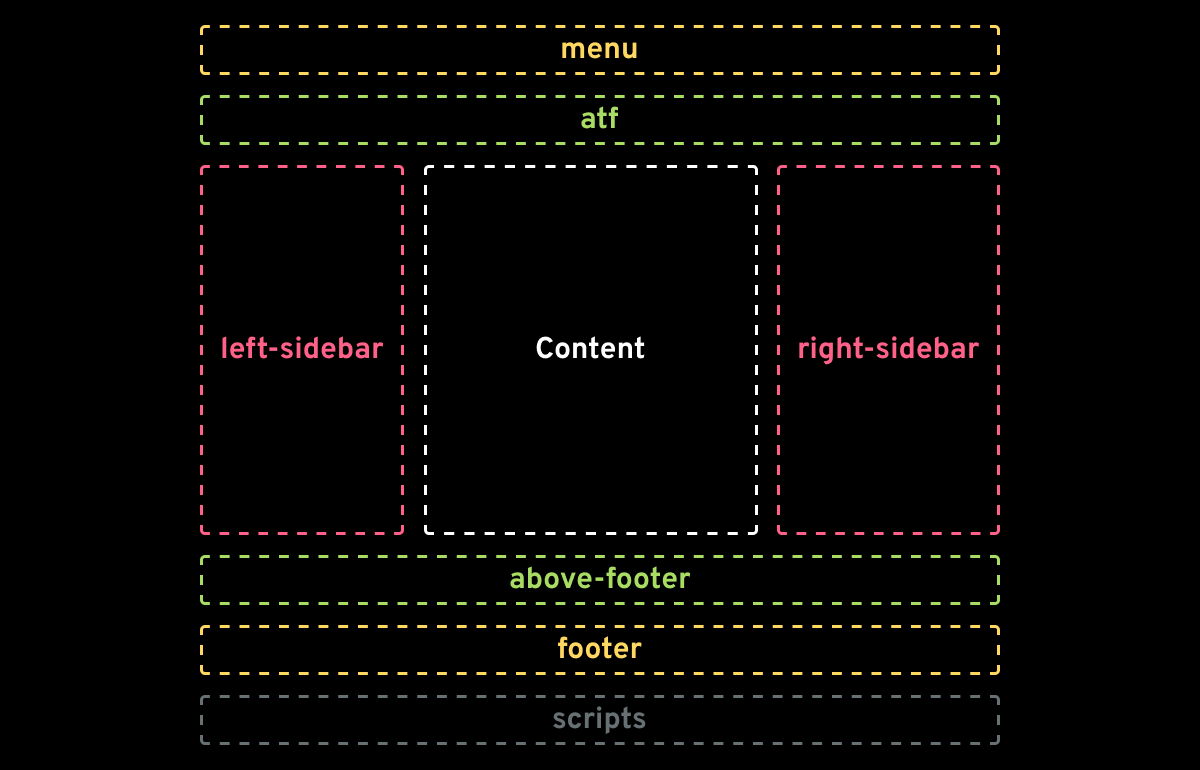
<Layout seo={seo} menu={menu} footer={footer}> <section slot="atf">...</section>
<aside slot="left-sidebar">...</aside> <main>Your main content</main> <aside slot="right-sidebar">...</aside>
<section slot="above-footer">...</section></Layout>
The menu
and footer
slots can be used to insert additional content inside your menu/footer. In case of Svelte, you can use snippets with the same names converted to camelCase
. The menu
and footer
keys are reserved for props in this case. You can use insideMenu
and insideFooter
instead:
<Layout {...layout}> {#snippet atf()} <div>ATF section</div> {/snippet}
<span>Your main content</span>
{#snippet insideFooter()} <div>Inserted inside the footer</div> {/snippet}</Layout>
For the React variant, you can pass the same names as props to the Layout
component. Any additional props passed to the component not listed above will be inserted directly on the body
tag.
Themes
To change the theme of your layout, you can extend the provided layout.scss
with the following line:
@use 'webcoreui/styles' as *;@use 'webcoreui/config' as *;@include setup(( fontRegular: '/fonts/Inter-Regular.woff2', fontBold: '/fonts/Inter-Bold.woff2' theme: 'light'));
API
type LayoutProps = { seo: SEOProps menu?: MenuProps footer?: FooterProps className?: string [key: string]: any}
type SvelteLayoutProps = { insideMenu?: Snippet atf?: Snippet leftSidebar?: Snippet rightSidebar?: Snippet aboveFooter?: Snippet insideFooter?: Snippet scripts?: Snippet children?: Snippet} & LayoutProps
type ReactLayoutProps = { insideMenu?: React.ReactNode atf?: React.ReactNode leftSidebar?: React.ReactNode rightSidebar?: React.ReactNode aboveFooter?: React.ReactNode insideFooter?: React.ReactNode scripts?: React.ReactNode children?: React.ReactNode} & LayoutProps
You can find more details about the different prop types on the following pages:
Prop | Purpose |
---|---|
seo | Sets SEO-related tags in the head for the page. |
menu | Sets the main navigation menu for the page. |
footer | Sets a footer for the page. |
className | Pass additional CSS classes for the wrapper of your main content. |
For Astro, you can use the above-mentioned slots to extend your layout with different sections. For Svelte and React components, you can define the following additional snippets (Svelte) and props (React):
Svelte & React Prop | Purpose |
---|---|
insideMenu | Sets additional HTML content inside the main navigation. |
atf | Sets additional HTML content below the main navigation and above the main content. |
leftSidebar | Adds a sidebar on the left-side next to the main content. |
rightSidebar | Adds a sidebar on the right-side next to the main content. |
aboveFooter | Sets additional content above the footer and below the main content. |
scripts | Sets scripts just before the closing of the body tag. |
children | Sets the main content. |