Device Mockup
Showcase content within the frame of devices
Use the DeviceMockup
component to display content in a mobile or desktop mockup. They help users visualize how content or designs will appear on actual devices, improving context and clarity.
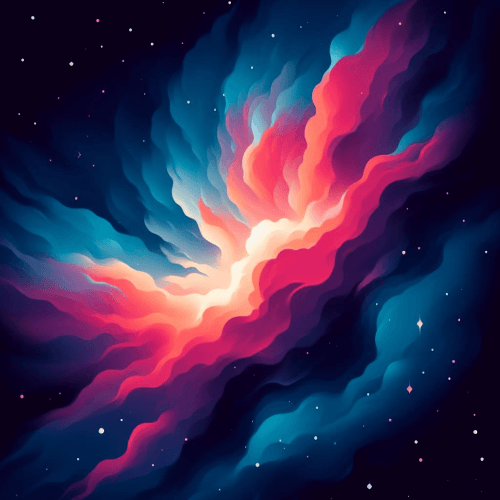
---import { AspectRatio, Icon } from 'webcoreui/astro'---
<DeviceMockup className="mobile-mockup"> <div class="grid sm"> <AspectRatio ratio="1/1.5"> <img src="/img/mockup.png" width="300" height="300" /> </AspectRatio> <Icon type="home" /> <span class="muted">Mobile Mockup</span> </div></DeviceMockup>
<style lang="scss" is:global> @use 'webcoreui/config' as *;
.mobile-mockup { @include spacing(auto-none); @include typography(center); @include background(primary-50);
svg { @include spacing(none-auto); }
max-width: 300px; }</style>
The DeviceMockup
block creates only the styled container of the device, you can use images and other elements to create your mockups. A common pattern is to use a single img
tag only that has all your mock details. You can use the AspectRatio
component to constrain the dimensions of your images.
Desktop
To turn your mobile device mockups into desktop mockups, you can set the type
prop on the component to desktop
:
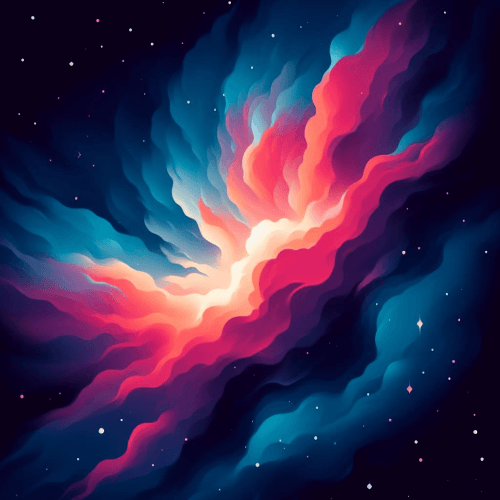
<DeviceMockup type="desktop"> <div class="grid sm"> <AspectRatio ratio="21:9"> <img src="/img/mockup.png" width="600" height="300" /> </AspectRatio> </div></DeviceMockup>
You can further customize your mockup using the following five props:
showButtons
: A flag to show/hide buttons in the top-left corner of the mockup. This is enabled by default.url
: A url or text that is displayed next to the buttons.closeButtonColor
: Set to customize the color of the first button.maximizeButtonColor
: Set to customize the color of the second button.minimizeButtonColor
: Set to customize the color of the third button.
webcoreui.dev
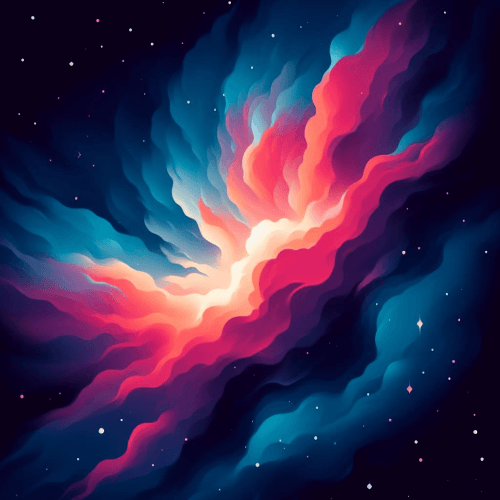
webcoreui.dev
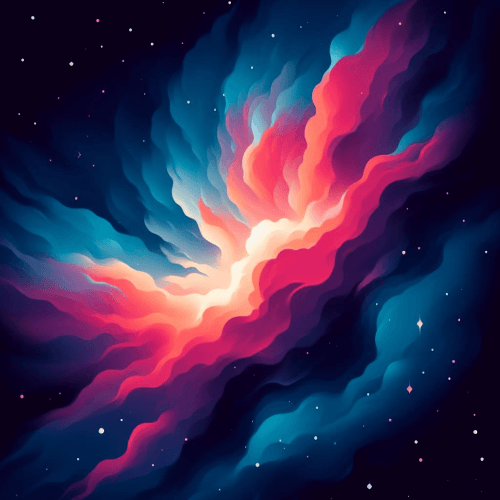
webcoreui.dev
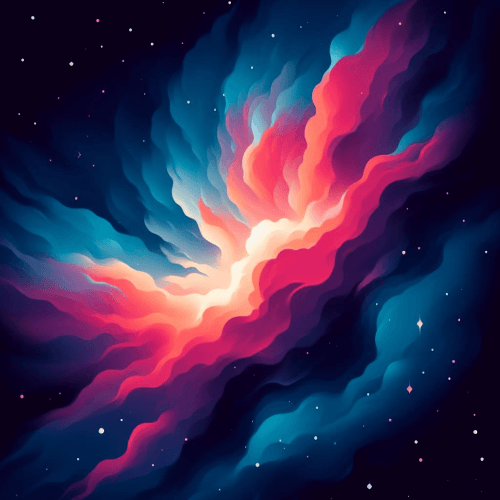
<DeviceMockup type="desktop" url="webcoreui.dev"> ...</DeviceMockup>
<DeviceMockup type="desktop" url="webcoreui.dev" showButtons={false}> ...</DeviceMockup>
<DeviceMockup type="desktop" url="webcoreui.dev" closeButtonColor="#D13824" maximizeButtonColor="#E7A121" minimizeButtonColor="#97E038"> ...</DeviceMockup>
API
type DeviceMockupProps = { type?: 'desktop' url?: string showButtons?: boolean closeButtonColor?: string maximizeButtonColor?: string minimizeButtonColor?: string className?: string}
Prop | Applicable type | Purpose |
---|---|---|
type | - | Set to "desktop" to change the style of your mockup. |
url | desktop | Sets a url or an arbitrary text for the address bar. |
showButtons | desktop | Shows action buttons on the top-left corner of the mockup. Defaults to true . |
closeButtonColor | desktop | Sets the color of the close button |
maximizeButtonColor | desktop | Sets the color of the maximize button |
minimizeButtonColor | desktop | Sets the color of the minimize button |
className | - | Pass additional CSS classes for your DeviceMockup component. |