Property Card and Carousel
Display property listings
Use the PropertyCard
component to display details of a property, such as real estate listings or rental units, in a concise and visually appealing way. The component can be configured in many different ways to get visually distinct elements.
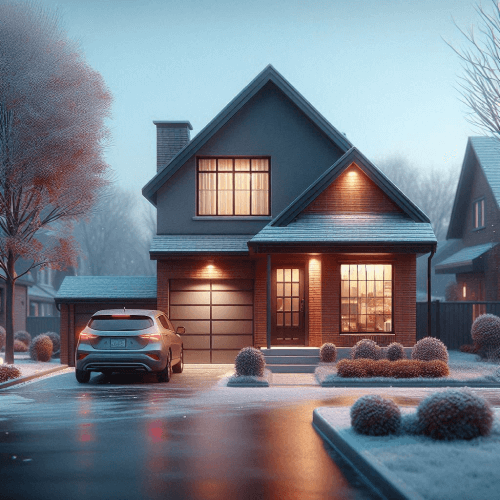
available for your exclusive rental.
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
---const propertyCardProps = { img: { src: '/img/property/property1.png', alt: '', height: 150, width: 500 }, ribbon: { text: 'Featured', theme: 'alert', offset: 25 }, rating: { score: 4 }, location: 'New Orleans', meta: 'Top rated', title: 'Elisabeth Apartman', buttons: [{ text: 'Check Availability', theme: 'info' }], amenities: [ 'Pool', 'Jacuzzi', 'Sauna', 'Free WiFi' ]}---
<PropertyCard {...propertyCardProps}> <span class="muted">Relax and unwind in this charming home...</span></PropertyCard>
The PropertyCard
component can be created in many different configurations using props. Only the img
and title
props are mandatory which sets an image for the property and a title.
Ribbons
To provide ribbons on the image, specify the ribbon
prop which expects an object with a text
property that sets the text for the ribbon. You can pass any valid RibbonProps
prop along to the object to customize the way your ribbon looks. To learn more about ribbons, read through the Ribbon
component documentation.
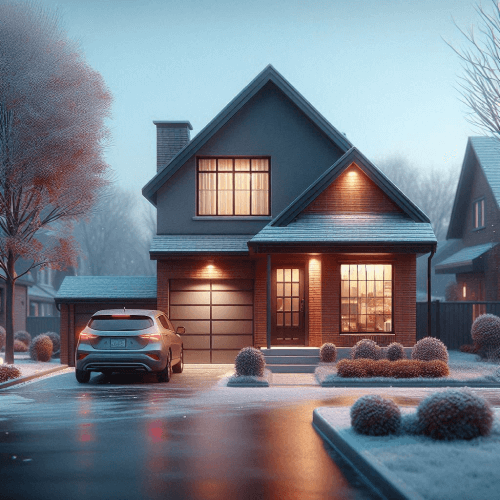
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
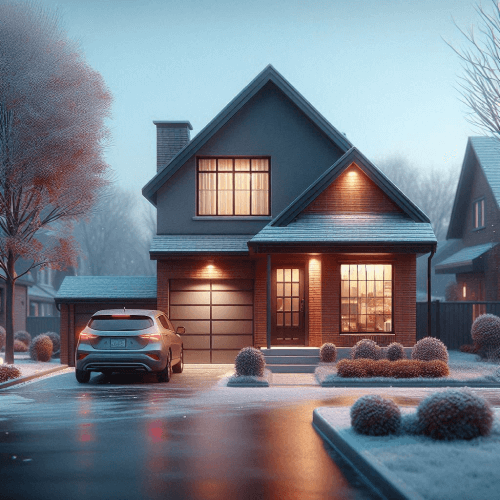
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
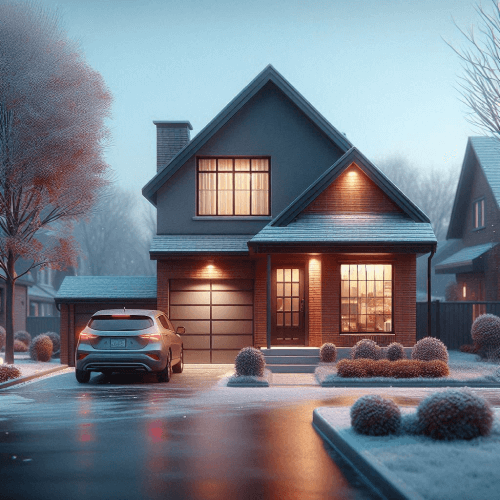
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
<PropertyCard {...propertyCardProps} ribbon={{ text: 'Featured', theme: 'warning', offset: 25 }}/>
<PropertyCard {...propertyCardProps} ribbon={{ text: 'Featured', theme: 'alert', type: 'folded' }}/>
Rating
Similar to the ribbon, the rating can also be customized using the Rating
component. For example, you can show the number of ratings or display a link to reviews in the following way:
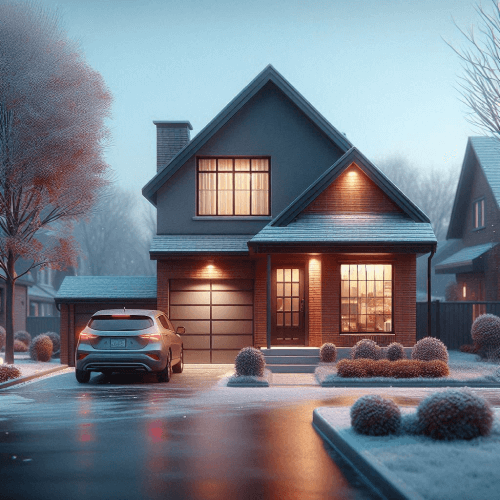
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
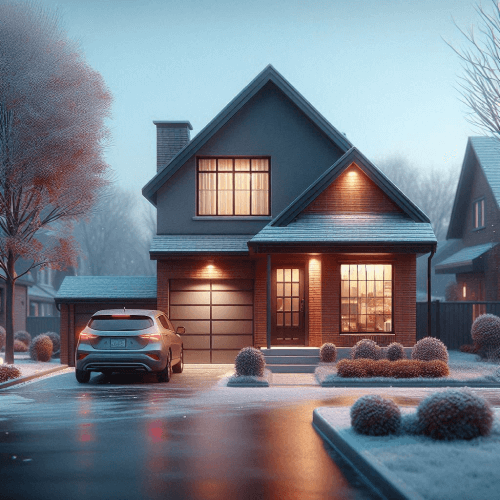
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
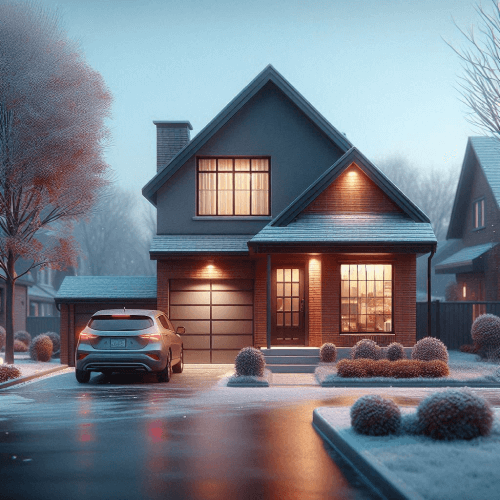
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
<PropertyCard {...propertyCardProps} rating={{ score: 4.4, text: '({0})', showEmpty: false, showText: true, reviewCount: 48 }}/>
<PropertyCard {...propertyCardProps} rating={{ score: 4.4, text: '({0})', reviewText: '{0} ratings', reviewLink: '#', showEmpty: false, showText: true, reviewCount: 48 }}/>
<PropertyCard {...propertyCardProps} rating={{ score: 4.4, showEmpty: false, showText: true, color: 'var(--w-color-warning)' }}/>
Meta information
The location
and meta
props can be used to add additional information next to the star rating:
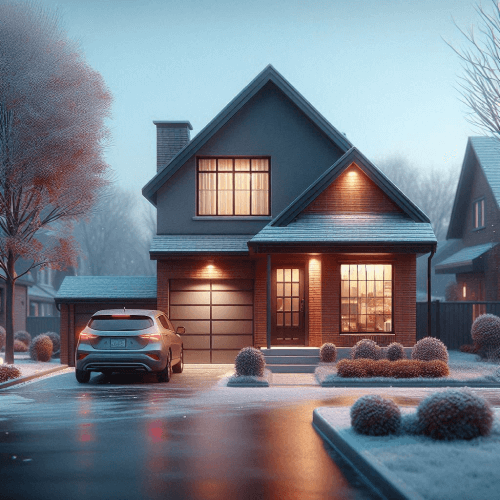
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
<PropertyCard {...propertyCardProps} location="New Orleans" meta="Downtown • Top rated"/>
CTAs
Providing a CTA is optional. The CTA prop accepts an array of Button
components, meaning you can also add multiple actions for users.
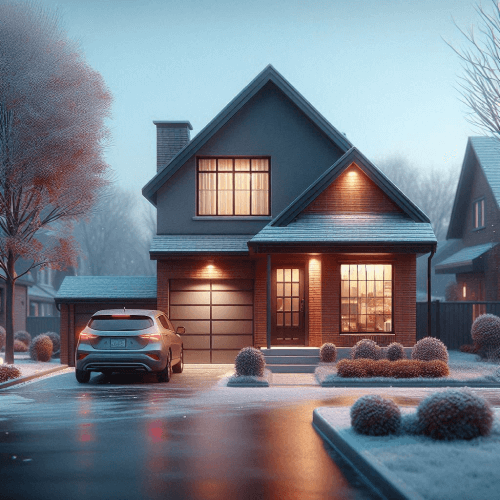
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
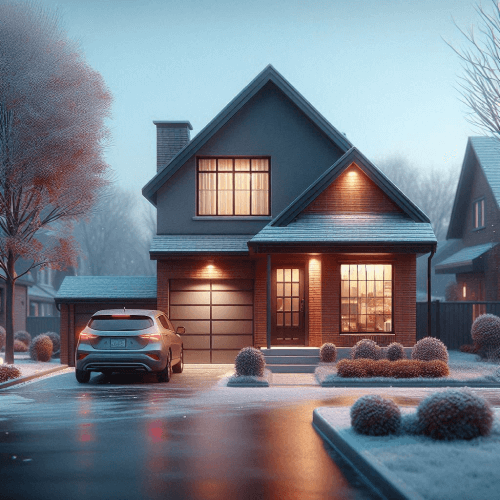
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
<PropertyCard {...propertyCardProps} buttons={[ { text: 'Check Availability' }, { text: 'Contact Owner', theme: 'outline' } ]}/>
buttons
prop, please read the API documentation of the Button
component. Main Content
The main content for the card can be defined as the children of the component. This means you can extend the card with additional components if needed, and build more complex UIs. For example, the following code adds a collapsible section for property owner details:
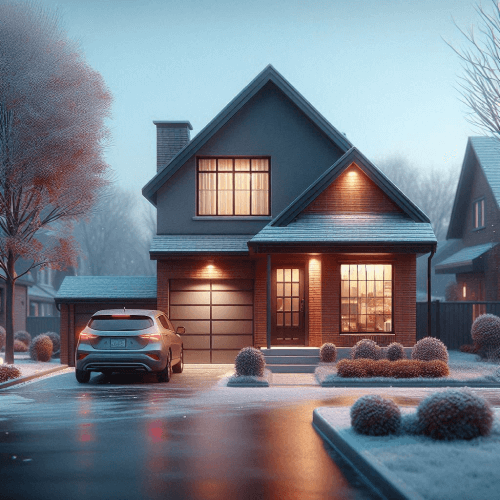
available for your exclusive rental.

Phone | +1 234 5678 |
emily@example.com |
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
---import { Avatar, Badge, Collapsible, Table } from 'webcoreui/astro'---
<PropertyCard {...propertyCardProps}> <div class="grid sm"> <span class="muted">Relax and unwind in this charming hom...</span> <Collapsible toggled={true} maxHeight="120px"> <div class="grid sm"> <div class="flex sm items-center"> <Avatar img="owner.png" size={50} /> <div class="flex column xxs"> <span>Emily</span> <span class="muted">Property Owner</span> </div> </div> <Table striped="row" offsetStripe={true} hover={true} data={[ ['Phone', '+1 234 5678'], ['Email', '<a href="#">emily@example.com</a>'] ]} /> </div>
<Badge slot="on" hover={true} theme="secondary">Show owner details</Badge> <Badge slot="off" hover={true} theme="secondary">Hide owner details</Badge> </Collapsible> </div></PropertyCard>
Amenities
Lastly, at the end of the card you can display a list of amenities using the amenities
array. This expects an array of strings:
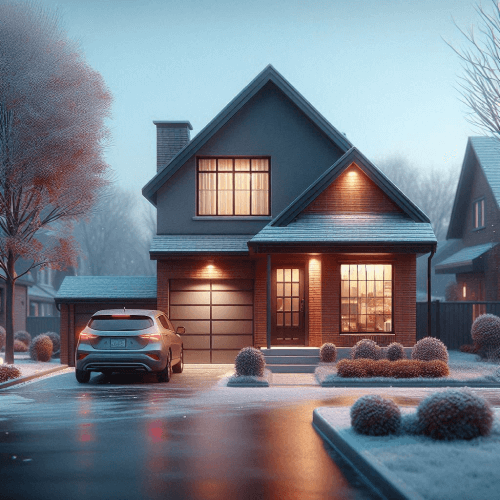
available for your exclusive rental.
-
Pool
-
Jacuzzi
-
Sauna
-
Free WiFi
<PropertyCard {...propertyCardProps} amenities={[ 'Pool', 'Jacuzzi', 'Sauna', 'Free WiFi' ]}/>
Property Carousels
You can combine the PropertyCard
component with the Carousel
component to also create property carousels in places with limited space:
---import { Carousel } from 'webcoreui/astro'
const properties = [...] // Your property objects---
<Carousel items={properties.length} itemsPerSlide={3} className="property-carousel"> {properties.map(property => ( <li> <PropertyCard {...property} /> </li> ))}</Carousel>
<style lang="scss" is:global> @use 'webcoreui/config' as *;
.property-carousel li { @include layout(h-start); }</style>
Carousel
component here. API
type PropertyCardProps = { img: { src: string alt: string width: number height: number lazy?: boolean } ribbon?: ({ text: string } & RibbonProps) rating?: RatingProps location?: string meta?: string title: string buttons?: ({ text: string icon?: IconProps['type'] | string } & ButtonProps)[] amenities?: string[] className?: string} & CardProps
You can find additional documentations below for the above types:
Prop | Purpose |
---|---|
img | Sets the property image for the card. |
img.src | Sets the path for the image. |
img.alt | Sets an alternate text for the image. |
img.width | Sets the width of the image. |
img.height | Sets the height of the image. |
img.lazy | Set to true to enable lazy loading for the image. |
ribbon | Sets a ribbon shown above the image. |
ribbon.text | Sets the text for the ribbon. |
rating | Sets the rating of the property. |
location | Sets a location for the property, shown under the title and image. |
meta | Sets additional text under the property's name. |
title | Sets a title for the property card. |
buttons | Sets CTA buttons under the rating. |
buttons.text | Sets the text for the CTA. |
buttons.icon | Sets an icon for the CTA. You can pass SVG strings, or an icon type for Astro components. |
amenities | Sets a list of amenities under the main content. |
className | Pass additional CSS classes for the PropertyCard component. |